In this hint, I will explain How to Get and Set a SharePoint Multiple Choice Field Value programmatically using C# SSOM Server Object Model?
Consider, you have a SharePoint List with a Choice Multiple Selection field and you would like to update and set this multiple choice Field Value programmatically using C#.
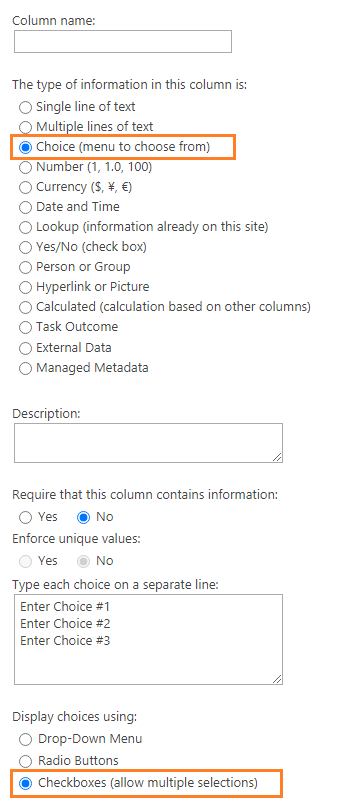
In this case, you should use the below “SetMultipleChoiceFieldValue()” function with your List name and field name to be able to set Multiple choice field value using C#.
C# Code
public void SetMultipleChoiceFieldValue() {
using(SPSite site = new SPSite(SPContext.Current.Site.Url)) {
using(SPWeb web = site.OpenWeb()) {
SPList list = web.Lists["List1"];
SPListItem item = list.GetItemById(1);
SPFieldMultiChoiceValue itemValue = new SPFieldMultiChoiceValue();
itemValue.Add("Choice 1");
itemValue.Add("Choice 2");
itemValue.Add("Choice 3");
item["FieldName"] = itemValue;
item.Update();
}
}
}
Note: the Field Class is SPFieldMultiChoice, and the Field Value Class is SPFieldMultiChoiceValue
In the previous section, we have learned how to set Choice Field Multiple Selection Value programmatically using C#, In this section, we will show how to get SharePoint Choice Field Multiple Selection Value Using C#.
C# Code
public void SetMultipleChoiceFieldValue() {
using(SPSite site = new SPSite(SPContext.Current.Site.Url)) {
using(SPWeb web = site.OpenWeb()) {
SPList list = web.Lists["List1"];
SPListItem item = list.GetItemById(1);
SPFieldMultiChoiceValue itemValue =
new SPFieldMultiChoiceValue(item["FieldName"].ToString());
foreach (string choice in itemValue)
{
// value is in choice
}
}
}
}
Conclusion
In conclusion, we have learned how to Get and Set a SharePoint Multiple Choice Field Value using Server Object Model C#?
Applies To
- SharePoint 2016.
- SharePoint 2013.
- SharePoint 2010.
- C# SSOM.
You may also like to read
- Get and Set a SharePoint Multiple Lookup Field Value Using Server Object Model C#.
- SharePoint Lookup Field Multiple Values Via C#.
- Get and Set a SharePoint Lookup Field Value Using Server Object Model C#.
- Get and Set a SharePoint URL Field Value Using Server Object Model C#.
- Get and Set a SharePoint Multiple Lookup Field Value Using Server Object Model C#.
- Retrieve a SharePoint Choice Field Value Using Server Object Model C#.
- Get and Set User Field SharePoint C#.
Have a Question?
If you have any related questions, please don’t hesitate to ask it at deBUG.to Community.
Pingback: Get SharePoint Choice Field Value In C# | SPGeeks
Pingback: Get URL value from Hyperlink Field in SharePoint using C# | SPGeeks