In this article, we will learn how to Auto Populate Field Values based on Lookup Selection In SharePoint 2016 Forms using JSOM and JQuery.
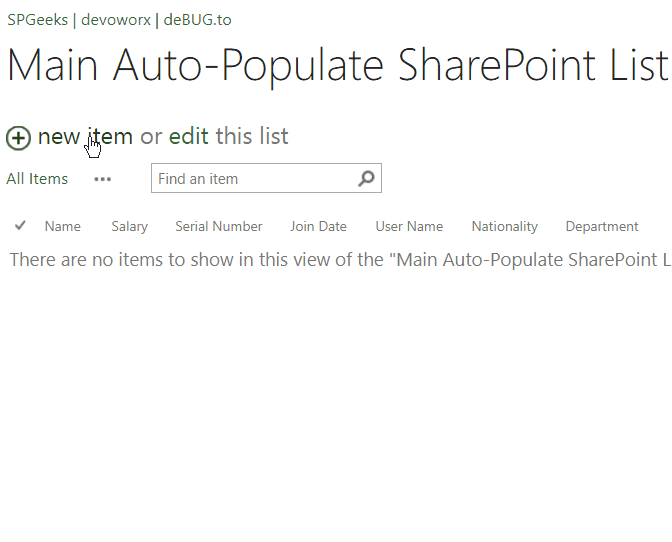
You might also like to read Auto Populate Column based on another Column on Text Change in SharePoint 2016 forms
To auto fill field values from another list based on Lookup Selection In SharePoint Forms, we’ll go through the following:
- 1 Auto Populate Field Values based on Lookup Selection In SharePoint
- 2 SharePoint Auto-Populate Column based on another Column on Text Change
-
3
Set field values from another list based on another selection
- 3.1 Auto Populate Text field value from another list based on lookup selection
- 3.2 Auto Populate Number field value from another list based on lookup selection
- 3.3 Auto Populate Date field value from another list based on lookup selection
- 3.4 Auto Populate Lookup field value from another list based on lookup selection
- 3.5 Auto Populate Choice field value from another list based on lookup selection
- 3.6 Auto Populate People field value from another list based on lookup selection
Auto Populate Field from another list based on a Selection
Consider, you have two lists, the first list has a column lookup field that holds data from the second List as shown below:
Main Auto Populate SharePoint List
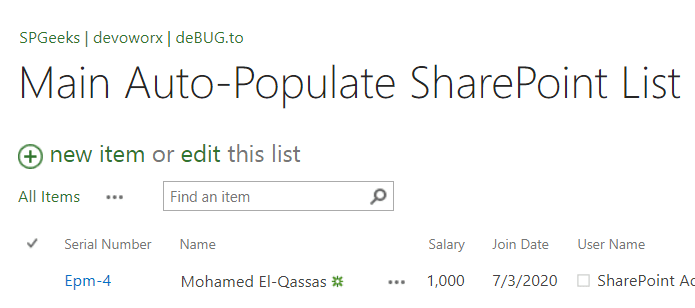
SharePoint Lookup List
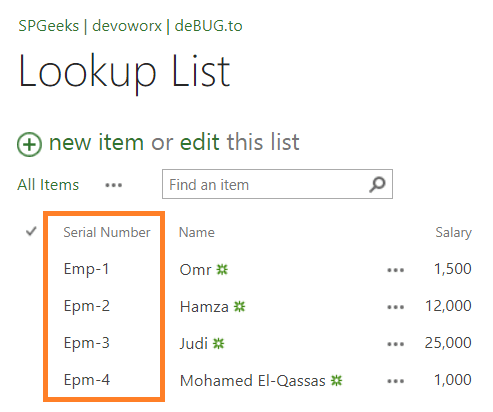
Now, you would like to fill field values from SharePoint Lookup list when you create a new item in the Main Auto Populate SharePoint List based on lookup selection as shown below:
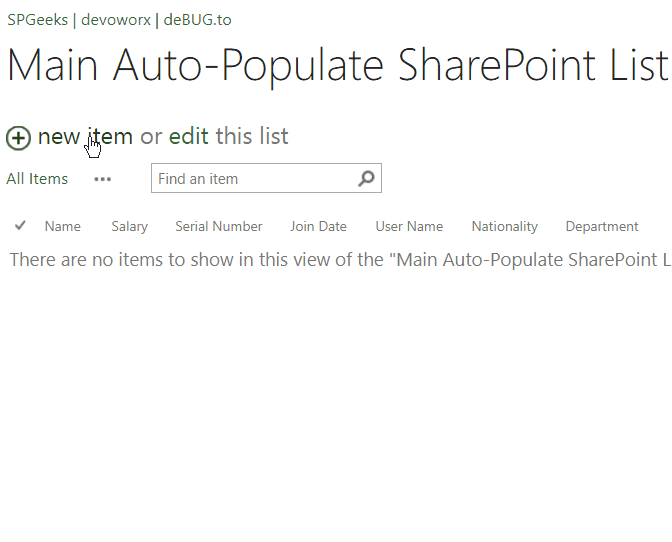
You might also like to read Using Lookup Field in Calculated Column SharePoint
Before we getting started, let’s first prepare the required fields that you need to get this code working properly.
- It’s recommended to download first the three list templates to get the structure of the same lists. this step is not mandatory but it helps you to test and understand the code in your side properly.
- As per your current lists, you should map the fields in your side with the corresponding fields in our list templates. don’t worry, we’ll try to help you to do that in this article.
Ex: in our code, we are using a “Serial Number” lookup field in the main list that reads the serial number values from the lookup list, so in your side, you must specify the corresponding lists and fields name correctly and replace these values in the code.
Please, if you stuck to use this code on your side, it’s recommended to ask your questions at deBUG.to to can help you faster.
Steps
- Open your first list (Main Auto-Populate SharePoint List).
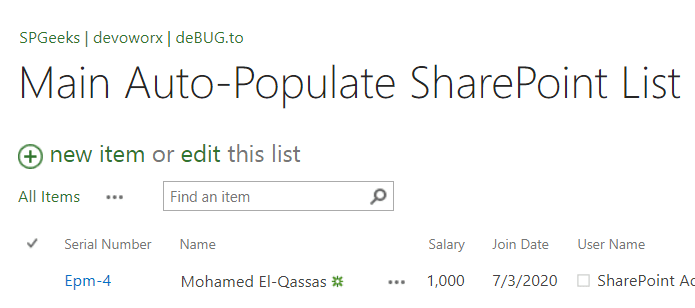
- Click on list tab > Form Web Part > Default New Form.
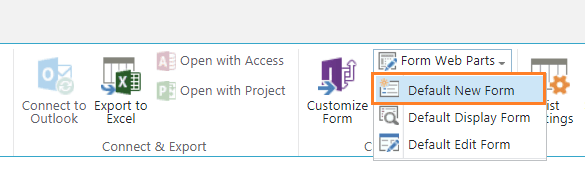
- The new form is now ready for edit, click on Add web part.
- Click on Media and Content > Add Script editor web part.
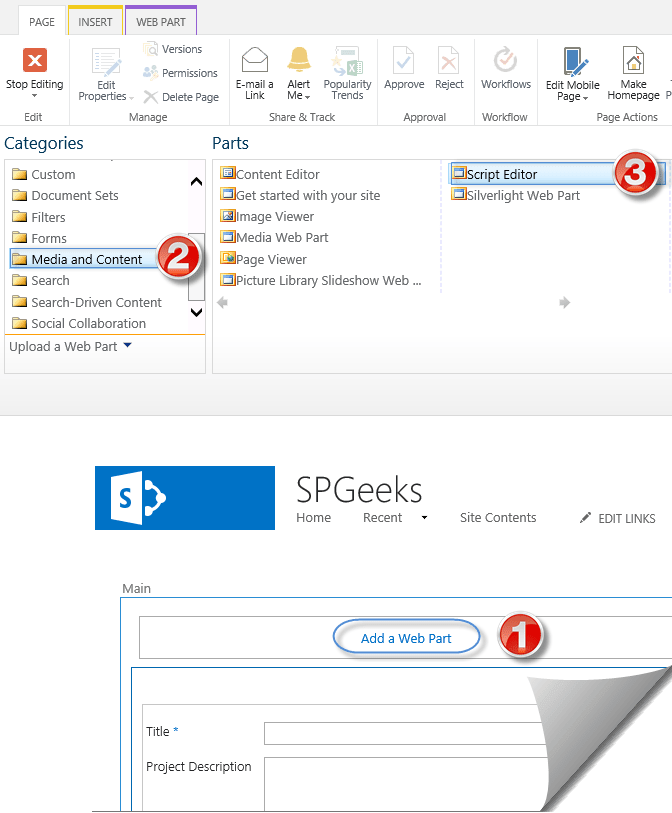
- Click on Edit Snippet.
- Download the JS code snippet on GitHub at Auto Populate Field Values based on Lookup Selection.
- Paste the downloaded code to Script Editor.
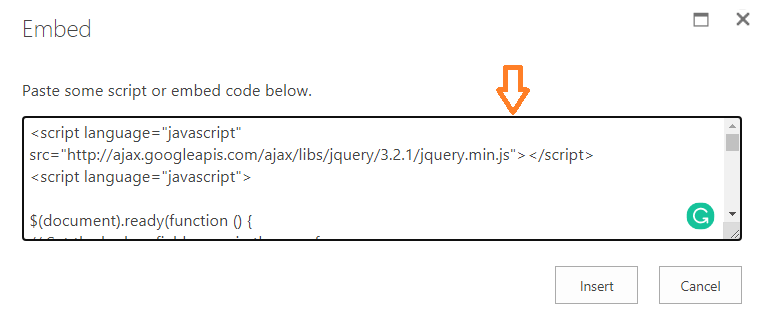
The code parts that you should change on your side
To get this code working properly on your side, you should do the following:
- Change the ‘Serial Number’ lookup field with the corresponding lookup field name in your list.
var LookupField= $("select[title='Serial Number']");
- Again, replace the lookup field name with your own, to get the selected lookup value.
var LookupField = $("select[title='Serial Number'] option:selected").text();
- Change the ‘Lookup List’ name with your own, it’s the second list, not the main list name.
var LookupList = clientContext.get_web().get_lists().getByTitle('Lookup List');
- Don’t forget to set the corresponding internal lookup field name in CAML Query specifically at “<fieldRef />” tag.
'<View><Query><Where><Eq> <FieldRef Name=\'Serial_x0020_Numaber\' /> <Value Type=\'Text\'>' + LookupField + '</Value></Eq></Where></Query><RowLimit>1</RowLimit></View>'
- In function “Succeed”, specifically in the below code, you should change
- The “Name” with the display field name in the new form in the Main List,
- The “Title” with the internal field name in the second Lookup List.
$("input[title='Name']").val(item.get_item('Title'));
Note: the field name that set in this line “Item.get_item(“Title”)” is the Internal Field Name, it’s not the displayed name, so if you have field Called for example “M Qassas” it should be “M_x0020_Qassas“
Alternatively, if you don’t like to use CAML query and you need to get Item details by ID, you can download and use this code at GitHub to get Item from another list by ID in SharePoint Using JSOM as shown below:

You might also like to check Auto-Populate Column from another list based on Text Change In SharePoint using JSOM If you would like to Auto Populate Field Values based on Lookup Field Selection instead of on Text change as shown below:
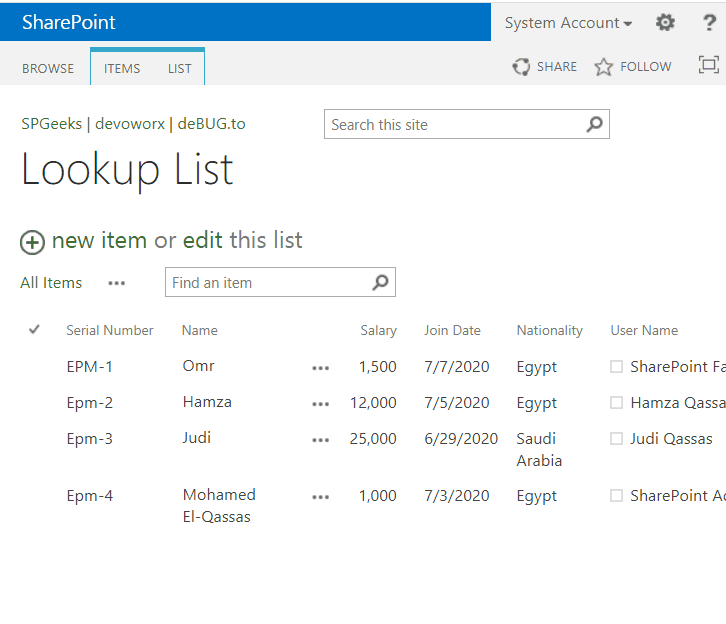
Set field values from another list based on another selection
In this section, we’ll concentrate on the code inside the “Succeed” function to learn how correctly set the field values based on its datatype like (Text, Number, Choice, Lookup, People).
function Succeed(sender, args) {
}
Auto Populate Text field value from another list based on lookup selection
To set a text field value from another list based on Lookup Selection using JQuery, you should use the below code
//Auto Fill Textfield value from another list based on the lookup selection
$("input[title='Field 1']").val(item.get_item('Field 2'));
In this code, you should set
- The value of the text field ‘Field 1‘ with the text field display name in your new form.
- The value of the text field ‘Field 2‘ with the internal name of the text field in the lookup list.
Now, when you select a specific value in the lookup field “Serial Number”, it will retrieve the related value of Field 2 and set it to the Field 1 “Name”.
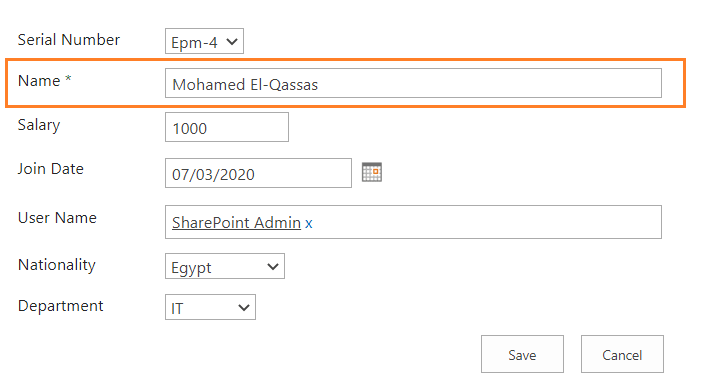
Auto Populate Number field value from another list based on lookup selection
To set a number field value from another list based on Lookup Selection using JQeury, you should use the below code:
//Auto Fill Number field value from another list based on the lookup selection
$("input[title='Field 1']").val(item.get_item('Field 2'));
In this code, you should set
- The value of the number field ‘Field 1‘ with the number field display name in your new form.
- The value of the number field ‘Field 2‘ with the internal name of the number field in the lookup list.
Now, when you select a specific value in the lookup field “Serial Number”, it will retrieve the related value of Field 2 and set it to the Field 1 “Salary”.
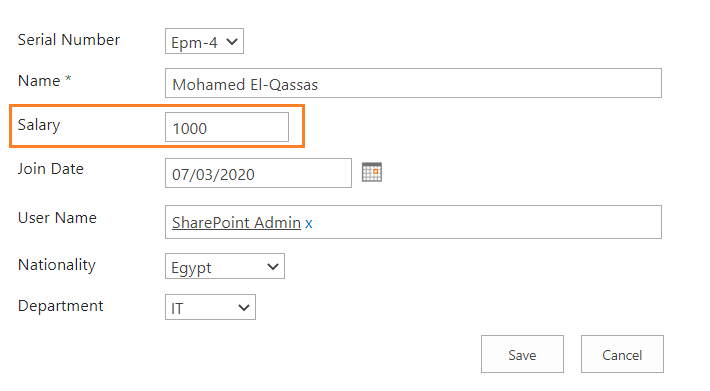
Auto Populate Date field value from another list based on lookup selection
To set a date field value from another list based on Lookup Selection using JQeury, you should use the below code:
//Auto Fill Date field value from another list based on the lookup selection
var joinDate = new Date(item.get_item('Field 2'));
var JoinDateFormat = joinDate.format("MM/dd/yyyy");
$("input[title='Field 1']").val(JoinDateFormat);
In this code, you should set
- The value of the date field ‘Field 1‘ with the date field display name in your new form.
- The value of the date field ‘Field 2‘ with the internal name of the date field in the lookup list.
Now, when you select a specific value in the lookup field “Serial Number”, it will retrieve the related value of Field 2 and set it to the Field 1 “Join Date”.
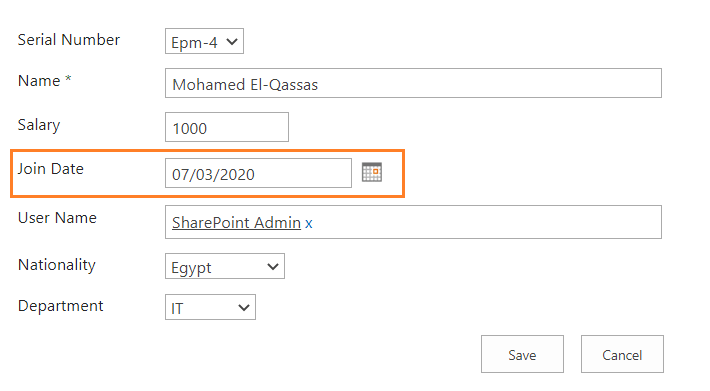
Auto Populate Lookup field value from another list based on lookup selection
To set a lookup field value from another list based on Lookup Selection using JQeury, you should use the below code:
//Auto Fill Lookup field value from another list based on the lookup selection
$("select[title='Field 1']").val(item.get_item('Field 2').get_lookupId());
In this code, you should set
- The value of the lookup field ‘Field 1‘ with the lookup field display name in your new form.
- The value of the lookup field ‘Field 2‘ with the internal name of the lookup field in the second lookup list.
Now, when you select a specific value in the lookup field “Serial Number”, it will retrieve the related value of Field 2 and set it to the Field 1 “Department”.
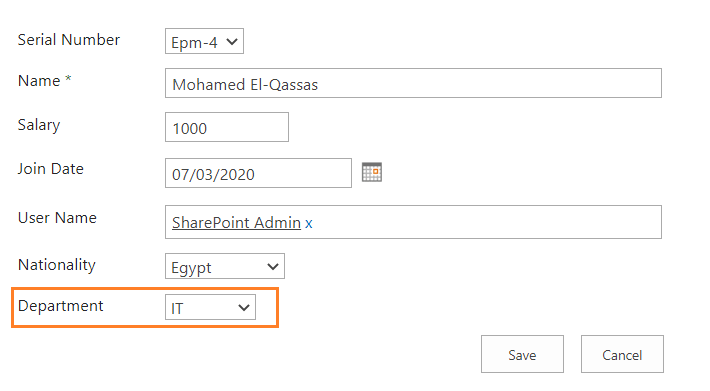
Auto Populate Choice field value from another list based on lookup selection
To set a choice field value from another list based on Lookup Selection using JQeury, you should use the below code:
//Auto Fill choice field value from another list based on the lookup selection
$("select[title='Field 1']").val(item.get_item('Field 2'));
In this code, you should set
- The value of the choice field ‘Field 1‘ with the choice field display name in your new form.
- The value of the choice field ‘Field 2‘ with the internal name of the choice field in the lookup list.
Now, when you select a specific value in the lookup field “Serial Number”, it will retrieve the related value of Field 2 and set it to the Field 1 “Nationality”.
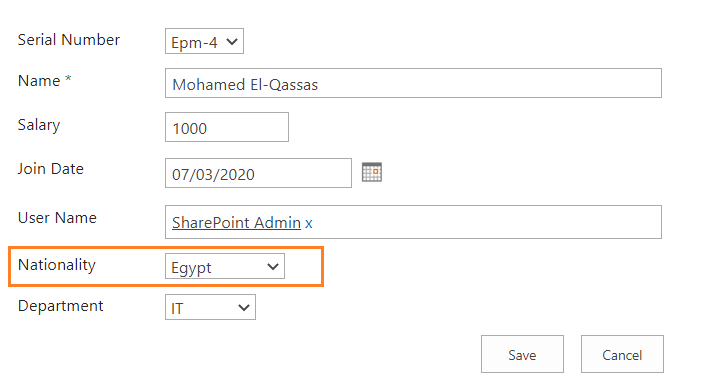
Auto Populate People field value from another list based on lookup selection
To set a People field value from another list based on Lookup Selection using JQeury, you should use the below code:
//Auto Fill People field value from another list based on the lookup selection
var context = SP.ClientContext.get_current();
var web = context.get_web();
var user = web.ensureUser(item.get_item('Field 2').get_lookupValue());
context.load(user);
context.executeQueryAsync(function(){
var form = $("table[class='ms-formtable']");
var userField = form.find("input[id$='ClientPeoplePicker_EditorInput']").get(0);
var peoplepicker = SPClientPeoplePicker.PickerObjectFromSubElement(userField);
// clear people Picker
while (peoplepicker.TotalUserCount > 0) {
peoplepicker.DeleteProcessedUser();
}
var loginName = user.get_loginName();
peoplepicker.AddUserKeys(loginName);},function(sender,args){ // on error
alert(args.get_message());});
In this code, you should set
- The value of the People field ‘Field 2‘ with the internal name of the People field in the lookup list.
Now, when you select a specific value in the lookup field “Serial Number”, it will retrieve the related value of Field 2 and set it to the first people field in your form.
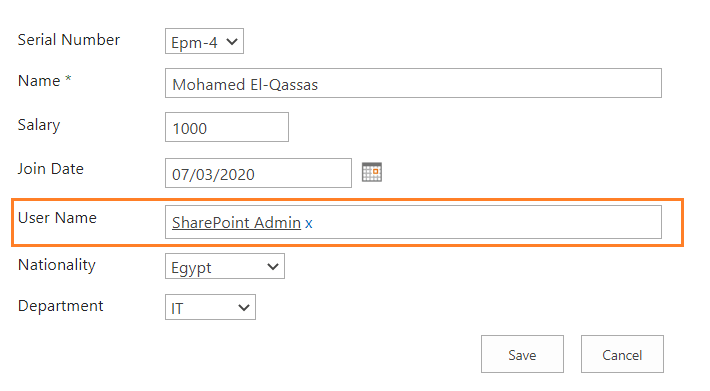
Please, if you stuck to use this code on your side, it’s recommended to ask your questions at deBUG.to to can help you faster.
Conclusion
In this article, we have explained How to auto populate SharePoint new form fields from another list based on the lookup field selected value using JSOM.
We have also explained how to
- Set People field value from another list based on Lookup Selection using JQeury.
- Set Lookup field value from another list based on Lookup Selection using JQeury.
- Set Date field value from another list based on Lookup Selection using JQeury.
- Set Choice field value from another list based on Lookup Selection using JQeury.
Applies To
- SharePoint 2019.
- SharePoint 2016.
- SharePoint 2013.
- SharePoint Online.
Download
Download the full code from GitHub at Auto Populate Field Values based on Lookup Selection, also Please, don’t forget to Follow Me to get the latest updates.
See Also
- Auto Serial Number in SharePoint New Forms using JSOM.
- Use SharePoint ID Field in Calculated Column.
- Disable SharePoint Multiline TextBox Field In Edit Form.
- Show and Hide Columns in SharePoint List Forms Using PowerShell.
Have a Question?
If you have any related questions, please don’t hesitate to ask it at deBUG.to Community.
Needs a https reference
Hello Mohamed El-Qassas,
Thank you for the wonderful tutorial. But something is not working for me can you please help me.
Scenario: I have a list called Student and in that all details are there like student name, address , dirstrict, city and country with mobile number.
Now in the form i have filed like student name and its binded to student list which will fetch only student name.
Now i require your help – If i select a student name in that drop down then all other fields in the form like address, district , city and country should fill up automatically.
Could you please suggest me modified code please as i am begginer to this env.
Hi Mohamed,
Thanks for a great tutorial. I’m a noobee to SharePoint online and i can’t figure out where to put the code. I tried following your instructions below, but I don’t see the second line where you say Click on list tab > Form Web Part > Default New Form. Can you help???
Open your first list.
Click on list tab > Form Web Part > Default New Form.
The new form is now ready for edit, click on Add web part.
Click on Media and Content > Add Script editor web part.
Click on Edit Snippet > add the below code.
Hi . I have 2 text boxes to upload from 2 separate lookups. I have changed the code to reference the necessary fields but it seems to only update the top dropdown (1st one). Where i select 1st dropdown or 2nd dropdown lookup. Any suggestions?
Hi, I need to access list in diferent context, like: var clientContext = new SP.ClientContext(“/Lists/”);
but in this case code returns “404 error not found”. If the source list and target list is on same subdomain and I use clientcontext.getCurrent(), it works.