In this hint, I will show How to get SharePoint Page Title and URL Programmatically?
using Microsoft.SharePoint;
void getSPTitle()
{
// to get the sharepoint page title
String Title= SPContext.Current.Item["Title"].ToString();
}
using Microsoft.SharePoint;
void getSPURL()
{
// to get the sharepoint page URL
String URL=System.Web.HttpContext.Current.Request.Path;
}
using Microsoft.SharePoint;
void getSPURL()
{
// to get the sharepoint page URL
SPContext.Current.Web.Url + "/" + SPContext.Current.File.Url;
}
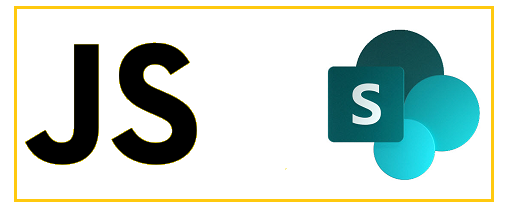
<script src ="http://ajax.googleapis.com/ajax/libs/jquery/1.9.1/jquery.min.js"> </script>
<script type = "text/javascript">
$(document).ready(function() {
// Wait until SP.JS has loaded before calling getWebUserData
ExecuteOrDelayUntilScriptLoaded(retrieveWebSite, "sp.js");
});
function retrieveWebSite() {
var clientContext = new SP.ClientContext(_spPageContextInfo.siteServerRelativeUrl);
this.oWebsite = clientContext.get_web();
clientContext.load(this.oWebsite);
clientContext.executeQueryAsync(
Function.createDelegate(this, this.onQuerySucceeded),
Function.createDelegate(this, this.onQueryFailed)
);
}
function onQuerySucceeded(sender, args) {
alert('Title: ' + this.oWebsite.get_title() +
' Description: ' + this.oWebsite.get_description());
}
function onQueryFailed(sender, args) {
alert('Request failed. ' + args.get_message() +
'\n' + args.get_stackTrace());
}
</script>
You might also like to check SharePoint 2016: Get Current User Using JavaScript
Conclusion
In conclusion, we have provided code samples to get SharePoint Page Title and URL Programmatically using JS and C#
Applies To
- SharePoint 2016.
- SharePoint 2013 / 2010.
- C# SSOM.
- JSOM.
You might also like to read
- Get and Set a SharePoint Multiple Lookup Field Value Using Server Object Model C#.
- SharePoint Lookup Field Multiple Values Via C#.
- Retrieve a SharePoint Choice Field Value Using Server Object Model C#.
Have a Question?
If you have any related questions, please don’t hesitate to Ask it at deBUG.to Community.
Pingback: Bind dropdown from SharePoint List in C# | SPGeeks
Pingback: Get and Set SharePoint Multiple Choice Field Value in C# | SPGeeks
this is very useful
Hello, after reading this awesome article i am too glad
to share my experience here with colleagues.
it’s proud for me to read my article
I’ve been surfing online more than three hours as of late, but I by no means found any fascinating article like yours. It’s beautiful
worth sufficient for me. In my opinion, if all website
owners and bloggers made just right content material as
you probably did, the internet shall be a lot more useful than ever before.
Surly your opinion so important for me 🙂 , and thank you for your comment 🙂