In this article, we will explain How to Get and Set SharePoint Lookup Field Programmatically using C#?
You might also like to read Using Lookup Field in Calculated Column SharePoint
In SharePoint List, you can create a column as a Lookup datatype that holds items from another list on the same site.
The Lookup columns (SPFieldLookup) can have multiple values or just single value based on the AllowMultipleValues property as shown below:
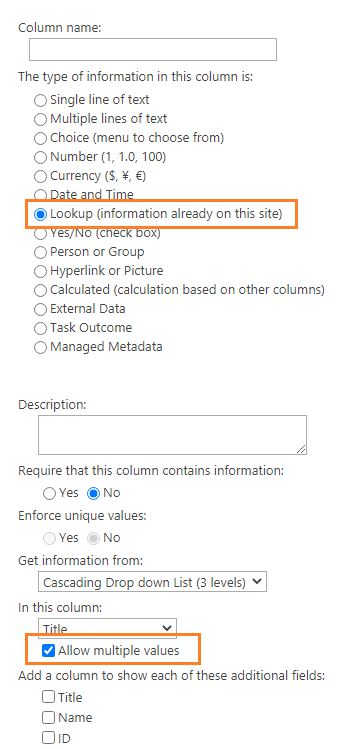
In case, the lookup column is a single value, the field value is an object of type SPFieldLookupValue with
- A 32-bit integer ID (LookupId) of the item in the list that the lookup field points to.
- A string value (LookupValue) of the field in the item that the lookup field points to.
In case, the lookup column allows multiple values, then the field value is an object of type SPFieldLookupValueCollection that is a collection of SPFieldLookupValue object.
So in this article, we will provide a complete Visual Studio Solution to learn How to Programmatically deal with Lookup field to to do the following:
- Set a SharePoint Lookup Field (Single Value) using C#.
- Set a SharePoint Lookup Field (Multiple Values) using C#.
- Get a SharePoint SharePoint Lookup Field (Single Value) using C#.
- Get a SharePoint SharePoint Lookup Field (Multiple Values) using C#.
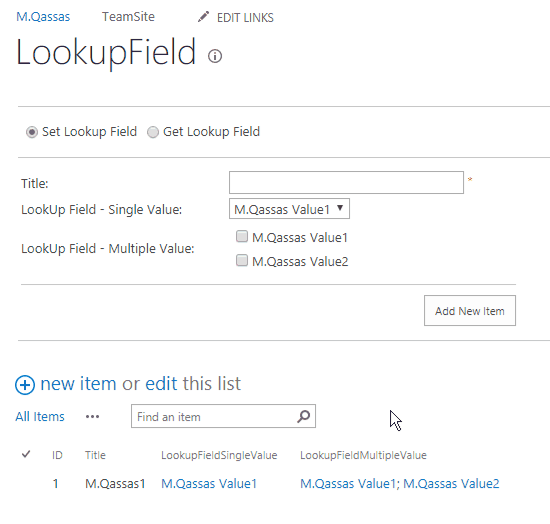
You can download the complete solution from GitHub at SharePoint Lookup Field Operations In SSOM C#
In this section, we we’ll learn how to get the Lookup Field Value (Single, Multiple) using C# as shown below:
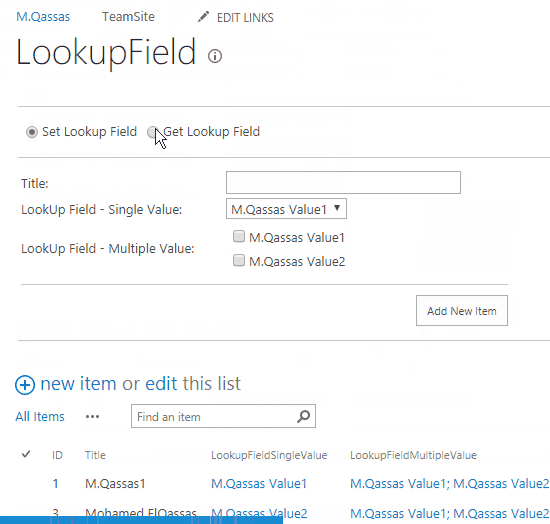
Get SPLookup Field (Single Value) Using C#
The below code show How to get a SP Lookup field (Single Values) based on an existing item in a SharePoint List.
using(SPSite site = SPContext.Current.Site) {
using(SPWeb web = site.OpenWeb()) {
// Get The SPList
SPList list = web.Lists["List Name"];
// Get a list item By ItemID
SPListItem item = list.GetItemById("A 32-bit integer that specifies the ID of List Item");
//Get Lookup Field - Single Value
SPFieldLookupValue SingleValue = new SPFieldLookupValue(item["The Lookup Field Name"].ToString());
int SPLookupID = SingleValue.LookupId;
string SPLookupValue = SingleValue.LookupValue;
}
}
Get SPLookup Field (Multiple Values) Using C#
The below code shows How to get the SharePoint Lookup field (Multiple Values) based on an existing item in a SharePoint List and bind the retrieved data to a drop-down list.
using(SPSite site = SPContext.Current.Site) {
using(SPWeb web = site.OpenWeb()) {
// Get The SPList
SPList list = web.Lists["LookupField"];
// Get a list item By ItemID
SPListItem item = list.GetItemById("A 32-bit integer that specifies the ID of List Item");
//Get Lookup Field - Multiple Values
SPFieldLookupValueCollection MultipleValues = item["The Lookup Field Name"] as SPFieldLookupValueCollection;
foreach(SPFieldLookupValue itemValue in MultipleValues) {
ListItem litem = new ListItem();
litem.Text = itemValue.LookupValue;
litem.Value = itemValue.LookupId.ToString();
dropdownlist.Items.Add(litem);
}
}
}
In this section, we we’ll learn how to update and set the Lookup Field Value (Single, Multiple) using C# as shown below:
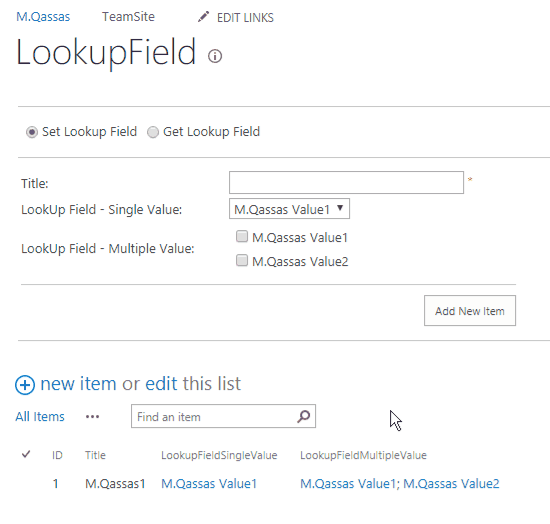
Set SPLookup Field (Multiple Values) Using C#
The below code helps you to set the SharePoint Lookup field (Multiple Values) based on an existing item in a SharePoint List.
using(SPSite site = SPContext.Current.Site) {
using(SPWeb web = site.OpenWeb()) {
// Allow Unsafe Updates to prevent the cross site scripting
web.AllowUnsafeUpdates = true;
// Get The SPList
SPList list = web.Lists["List Name"];
// Add a new list item
SPListItem item = list.Items.Add();
// Set the default Title field
item["Title"] = "string value";
//Set Lookup Field - Multiple Values
SPFieldLookupValueCollection itemValues = new SPFieldLookupValueCollection();
foreach(ListItem litem in your collection) {
itemValues.Add(new SPFieldLookupValue("A 32-bit integer that specifies the ID of the lookup field", "A string that contains the value of the lookup field"));
}
item["The Lookup Field Name"] = itemValues;
// Submit your Item
item.Update();
web.AllowUnsafeUpdates = false;
}
}
Set SPLookup Field (Single Value) Using C#
The below code show How to set SharePoint Lookup field (Single Values) based on an existing item in a SharePoint List.
using(SPSite site = SPContext.Current.Site) {
using(SPWeb web = site.OpenWeb()) {
// Allow Unsafe Updates to prevent the cross site scripting
web.AllowUnsafeUpdates = true;
// Get The SPList
SPList list = web.Lists["List Name"];
// Add a new list item
SPListItem item = list.Items.Add();
// Set the default Title field
item["Title"] = "String Value";
//Set the Lookup Field - Single Value
item["The Lookup Field Name"] = new SPFieldLookupValue("A 32-bit integer that specifies the ID of the lookup field", "A string that contains the value of the lookup field");
// Submit your Item
item.Update();
web.AllowUnsafeUpdates = false;
}
}
Conclusion
In conclusion, we have learned How to work with SharePoint Lookup Field Programmatically to to do the following:
- Set a SharePoint Lookup Field (Single Value) using C#.
- Set a SharePoint Lookup Field (Multiple Values) using C#.
- Get a SharePoint SharePoint Lookup Field (Single Value) using C#.
- Get a SharePoint SharePoint Lookup Field (Multiple Values) using C#.
Download
You can download the complete solution from
- GitHub: SharePoint Lookup Field Operations using SSOM C#.
- TechNet: SharePoint Lookup Field Operations In SSOM C#.
You might also like to read
- Bind dropdown from SharePoint List in C#.
- Get and Set a SharePoint URL Field Value Programmatically.
- Get and Set User Field SharePoint C#.
- How to get the Title and URL of SharePoint Page via Object Model?
- Get and Set a SharePoint Multiple Choice Field Value Using Server Object Model C#.
- Retrieve a SharePoint Choice Field Value Using Server Object Model C#.
Have a Question?
If you have any related questions, please don’t hesitate to ask it at deBUG.to Community.
Pingback: Get SharePoint Choice Field Value In C# | SPGeeks
Pingback: Bind dropdown from SharePoint List in C# | SPGeeks
Pingback: Get and Set SharePoint Multiple Choice Field Value in C# | SPGeeks
Pingback: Get and Set SharePoint User Field Value in C# | SPGeeks
Pingback: Get URL value from Hyperlink Field in SharePoint using C# | SPGeeks